npm install -g npm@4
Wednesday, December 20, 2017
Friday, December 15, 2017
Bluetooth keyboard shortcut on iOS 11
Spotlight search.
Change keyboard language.
Go to home.
Volume up/down.
Mute Volume.
CMD + Space
Change keyboard language.
CTRL + Space
Go to home.
CMD + H
Volume up/down.
F12/F13
Mute Volume.
F11
Wednesday, December 13, 2017
Align center tab in Ant Design
Add next style option to the tab component.
tabBarStyle={{textAlign: 'center'}}
100% height in Ant Design
In ant design you want to 100% height of some component.
Add next style option.
Add next style option.
style={{height:"100vh"}}
Thursday, December 7, 2017
Add permanently ssh key on macOS
Run next command.
Add config file in "~/.ssh/" with next contents.
Key option is AddKeysToAgent, UseKeychain.
ssh-add -K ~/.ssh/[YOUR_PRIVATE_KEY]
Add config file in "~/.ssh/" with next contents.
Host github.com
HostName github.com
User enzinier
PreferredAuthentications publickey
IdentityFile /Users/jason/.ssh/enzinier-GitHub
AddKeysToAgent yes
UseKeychain yes
Key option is AddKeysToAgent, UseKeychain.
Monday, September 18, 2017
Debugging react app with Jetbrain WebStorm
If you try debugging react app with JetBrains WebStorm, you nothing to do.
For debugging you must install JetBrains IDE Support chrome extension.
JetBrains IDE Support
For debugging you must install JetBrains IDE Support chrome extension.
JetBrains IDE Support
Tuesday, April 4, 2017
Get parameter from url
function getUrlParameter(paramName) {
var currentUrl = window.location.search.substring(1);
var urlParams = currentUrl.split("&");
for (var i = 0; i < urlParams.length; i++) {
var paramNames = urlParams[i].split("=");
if (paramNames[0] == paramName) {
return paramNames[1];
}
}
}
Wednesday, March 29, 2017
Permission denied issue on yarn global package
Unlike npm, location of global package on yarn is "/usr/bin" as default.
Therefore running yarn command without sudo occur to deny permission.
Avoid this situation, follow next instruction.
Therefore running yarn command without sudo occur to deny permission.
Avoid this situation, follow next instruction.
Check your default bin location of yarn.
yarn global bin
Create new directory in your favorite location.
mkdir ~/.yarn/global
Change default bin location of yarn.
yarn config set prefix ~/.yarn/global
And add path as environment variable in "~/.bashrc".
export PATH=${PATH}:`yarn global bin`
Saturday, March 25, 2017
Ubuntu | "/sbin/ldconfig.real: /usr/lib/nvidia-375/libEGL.so.1 is not a symbolic link"
Run next commands.
sudo mv /usr/lib/nvidia-375/libEGL.so.1 /usr/lib/nvidia-375/libEGL.so.1.org
sudo mv /usr/lib32/nvidia-375/libEGL.so.1 /usr/lib32/nvidia-375/libEGL.so.1.org
sudo ln -s /usr/lib/nvidia-375/libEGL.so.375.39 /usr/lib/nvidia-375/libEGL.so.1
sudo ln -s /usr/lib32/nvidia-375/libEGL.so.375.39 /usr/lib32/nvidia-375/libEGL.so.1
Sunday, March 5, 2017
"The user limit on the total number of inotify watches was reached"
This error reach a system limit on the number of files you can monitor.
See your current system limit.
See your current system limit.
cat /proc/sys/fs/inotify/max_user_watches
You can temporarily change limit with next command.
sudo sysctl fs.inotify.max_user_watches=524288
sudo sysctl -p
Also you can permanently change limit with next command.
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf
sudo sysctl -p
Saturday, March 4, 2017
copy and paste to other file in neovim
Open source file and press A key for select range.
Then press "+yy.
Open destination file and press "+p at your favorite location.
Then press "+yy.
Open destination file and press "+p at your favorite location.
How to add shortcut in launcher on Ubuntu 16.04 LTS
Shortcut files of launcher are placed in ~/.local/share/applications.
Create .desktop file to reference next code.
Create .desktop file to reference next code.
Friday, February 24, 2017
Sunday, February 19, 2017
How to setup GKE?
I'm usually manage google cloud command in Postman.
Follow next orders.
Follow next orders.
-
Create own network.
curl -X POST -H "Content-Type: application/json" -H "Authorization: {{Your Access Token}}" -H "Cache-Control: no-cache" -d '{ "name": "{{Your Network Name}}", "description": "{{Your Network Description}}", "autoCreateSubnetworks": false }' "https://www.googleapis.com/compute/v1/projects/{{Your Project ID}}/global/networks"
-
Create subnetwork.
curl -X POST -H "Content-Type: application/json" -H "Authorization: {{Your Access Token}}" -H "Cache-Control: no-cache" -d '{ "ipCidrRange": "10.10.0.0/20", "name": "asia-east1", "network": "https://www.googleapis.com/compute/v1/projects/{{Your Project ID}}/global/networks/{{Your Network Name}}" }' "https://www.googleapis.com/compute/v1/projects/{{Your Project ID}}/regions/asia-east1/subnetworks"
-
Create cluster in GKE.
curl -X POST -H "Content-Type: application/json" -H "Authorization: {{Your Access Token}}" -H "Cache-Control: no-cache" -H "Postman-Token: bc7eddb2-be25-29f6-9af4-be948e1519b6" -d '{ "cluster": { "name": "{{Your Cluster Name}}", "zone": "asia-east1-a", "network": "{{Your Network Name}}, "loggingService": "logging.googleapis.com", "monitoringService": "none", "description": "{{Your Cluster Description}}", "subnetwork": "asia-east1", "nodePools": [ { "initialNodeCount": 1, "config": { "machineType": "n1-standard-2", "imageType": "GCI", "diskSizeGb": 100, "localSsdCount": 1, "oauthScopes": [ "https://www.googleapis.com/auth/compute", "https://www.googleapis.com/auth/devstorage.read_write", "https://www.googleapis.com/auth/bigquery", "https://www.googleapis.com/auth/sqlservice.admin", "https://www.googleapis.com/auth/datastore", "https://www.googleapis.com/auth/logging.write", "https://www.googleapis.com/auth/monitoring.write", "https://www.googleapis.com/auth/bigtable.data", "https://www.googleapis.com/auth/servicecontrol", "https://www.googleapis.com/auth/service.management.readonly", "https://www.googleapis.com/auth/trace.append" ] }, "autoscaling": { "enabled": false }, "management": { "autoUpgrade": false, "autoRepair": false, "upgradeOptions": {} }, "name": "{{Your Pool Name}}" } ], "masterAuth": { "username": "admin" } } }' "https://container.googleapis.com/v1/projects/{{Your Project ID}}/zones/asia-east1-a/clusters"
-
Get credential of cluster.
gcloud container clusters list
gcloud config set container/cluster {{Your Cluster Name}}
gcloud config set compute/zone {{Your Zone ID}}
echo "export GOOGLE_APPLICATION_CREDENTIALS={{Your Key File Address}}" >> ~/.bash_profile
gcloud container clusters get-credentials {{Your Cluster Name}}
Wednesday, February 15, 2017
mysql data directory error on kubernetes.
If you meet next error...
[ERROR] --initialize specified but the data directory has files in it. Aborting.
You should add next argument in recipe.
spec:
containers:
- name: db-mysql
image: mysql:5.7
args: ["--ignore-db-dir=lost+found"]
Monday, February 13, 2017
Private docker registry on kubernete recipe
Encrypt docker config to base64.
cat ~/.docker/config.json | base64
Add encrypted data to data element.
apiVersion: v1
kind: Secret
metadata:
name: docker-config
type: kubernetes.io/dockerconfigjson
data:
.dockerconfigjson: ewoJI...
Refer docker-config as same level with container in deployment.
imagePullSecrets:
- name: docker-config
Sunday, February 12, 2017
Can't see console log of react native in nuclide.
Go to nuclide setting and change value of "Tag to include" to "^(.*)$" like this.
Friday, February 10, 2017
Connect to localhost inside docker
If your service inside docker want to connect to localhost, you can attach next option.
docker run --net="host"
Monday, January 23, 2017
Install KakaoTalk on Ubuntu 16 LTS
Run next command.
sudo apt-get install wine playonlinux fonts-nanum*
sudo apt-get install wine playonlinux fonts-nanum*
Keep clicking Next button.
Select "Install a program in a new virtual drive" and click next button.
Type name of virtual drive anything.
Check option like next screenshot.
Select "Install a program in a new virtual drive" and click next button.
Type name of virtual drive anything.
Check option like next screenshot.
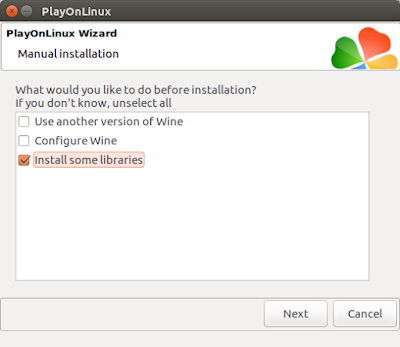
Select option "32 bits windows application".
Check next libraries.
Check next libraries.
- POL_Install_gdiplus
- POL_Install_gecko (For korean font)
- POL_install_riched30 (For korean font)
- POL_install_wmp10 (For alert)
- POL_install_wmpcodecs (For alert)
Then keep going click next button.
Don't check "Run kakaotalk".
In last, you can create shortcut for kakaotalk application.
Don't check "Run kakaotalk".
In last, you can create shortcut for kakaotalk application.
Saturday, January 21, 2017
Google Storage By Java
If you were deleted all files in folder then folder also deleted.
final Storage storage = StorageOptions.getDefaultInstance().getService();
boolean succeed = storage.delete(BlobId.of("bucket-name", "1/lenna.jpg"));
if (succeed) {
// File was deleted.
}
else {
// File not found!
}
ref. https://cloud.google.com/appengine/docs/flexible/java/using-cloud-storage
Google Cloud Authorization
Environment: OS X El Capitan
Use default credential or google cloud authorization.
Install google-cloud-sdk.
Authorize google cloud.
ref. https://developers.google.com/identity/protocols/application-default-credentials
Use default credential or google cloud authorization.
Install google-cloud-sdk.
brew cask install google-cloud-sdk
Authorize google cloud.
gcloud auth application-default login
ref. https://developers.google.com/identity/protocols/application-default-credentials
Subscribe to:
Posts (Atom)